The Friday Fragment
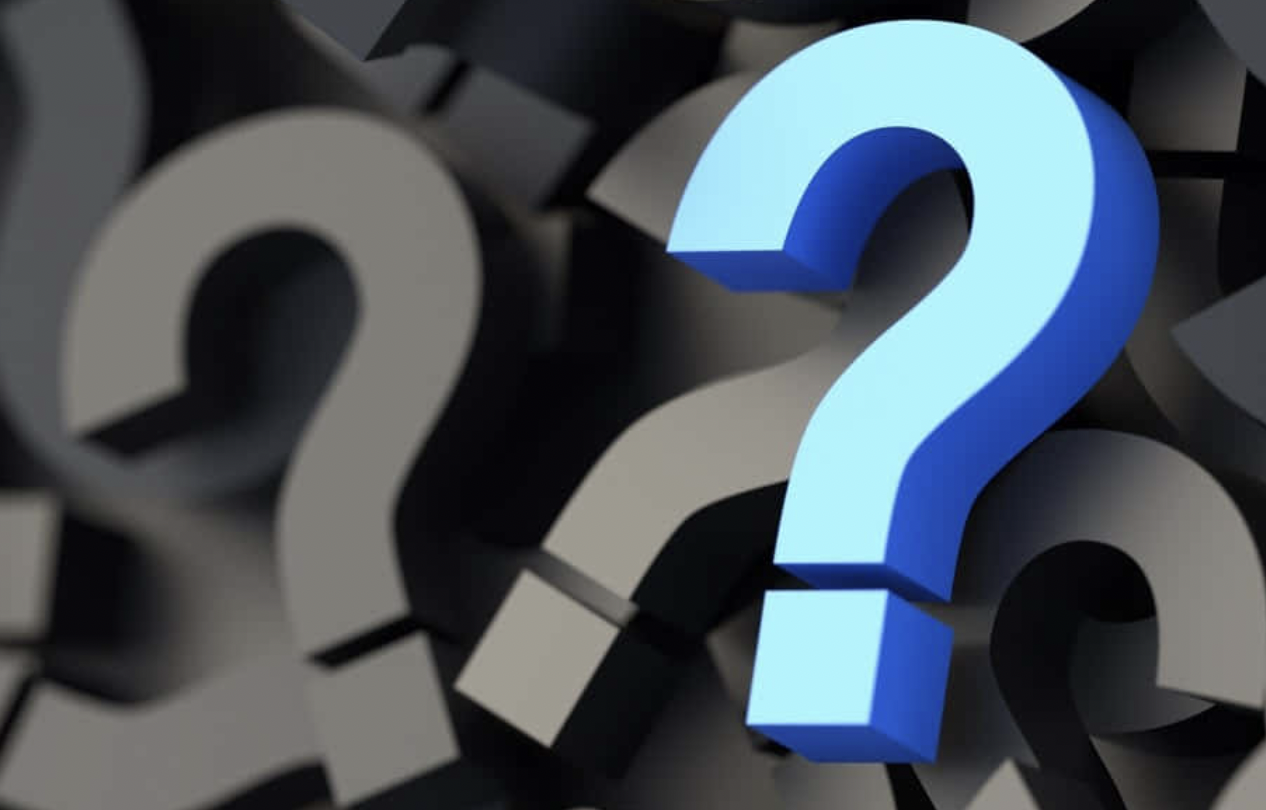
It's Friday, and time again for the Friday Fragment: our weekly programming-related puzzle.
This Week's Fragment
Some future Friday Fragments will make use of web services. So we'll take it one step at a time, starting this week:
Code a simple REST web service that, when called via GET, will randomly answer rock, paper, or scissors.
Your result can be fancy (wrapped in XML or JSON syntax), or simple: just the word. To “play along”, provide either the URL or the code for the solution. You can post it as a comment or send it via email.
Last Week's Fragment - Solution
Last week's puzzle was to code a happy number finder. A happy number is a positive integer which you can replace by the sum of the squares of its digits and repeat the process until you get to 1. For example, 7 is a happy number because its series ends at 1: 72 = 49, 42 + 92 = 97, 92 + 72 = 130, 12 + 32 + 02 = 10, 12 + 02 = 1.
If you tried this, you know it's not only a study in happy numbers, but also happy cases. The basic solution is easy; I coded a quick inductive solution in Ruby as an extension to the Integer class:
def is_happy
return case
when self == 1 then true
when self < 1 then false
else self.digits.inject(0) { | sum, ea | (ea * ea) + sum }.is_happy
end
end
That's nice and simple, and 0.is_happy, 1.is_happy, and 7.is_happy all work swimmingly. However, 2.is_happy loops forever until crashing with a stack overflow. The reason is that the sum of squares of digits calculations for unhappy numbers run in infinite cycles (perhaps that's why they're unhappy). We can prevent that with a cache of numbers we've seen before:
@@cache = Hash.new
def is_happy
return @@cache[self] if @@cache.include?(self)
@@cache[self] = false
result = case
when self == 1 then true
when self < 1 then false
else self.digits.inject(0) { | sum, ea | (ea * ea) + sum }.is_happy
end
@@cache[self] = result
return result
end
I posted the complete code as a comment to last week's puzzle.
No one sent a solution, so I'll keep the 2011 Corvette ZR1 prize to myself. But give this week's puzzle a try; fortune or fame (or at least happiness) may await you.