The Friday Fragment
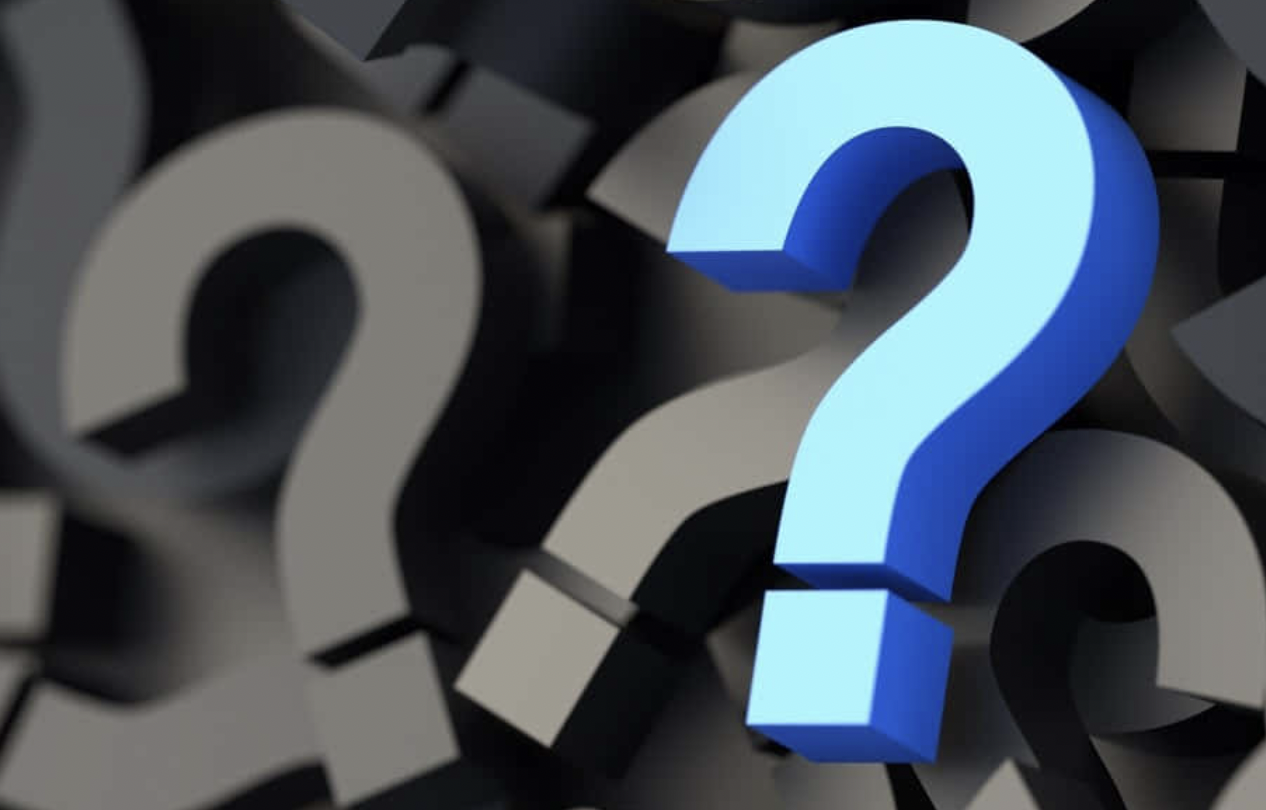
It's Friday, and time again for the Friday Fragment: our weekly programming-related puzzle.
This Week's Fragment
Let's do another game bot: this time, just a bit more sophisticated than randomly answering rock, paper, or scissors:
Code a simple REST web service to play tic-tac-toe. It should accept a nine-character string representing the current "game board" with space, X, or O in each position (starting from the top left, going left-to-right and down). It should return a nine-character string with your next move added.
For example, the game board at left is represented by the string " O X OX ". X goes first; if you receive all spaces (empty board), go first with X. Otherwise, take the next move: if you receive an even number of Xs and O's, replace a space with an X; if you receive an odd number of plays, replace a space with an O. And no cheating: if you do anything other than take the next legal move, you lose.
This is your chance to have a computer play your games for you. Can you code something that plays as wisely as you would?
To “play along,” provide either the URL or the code for the solution. You can post it as a comment or send it via email. We'll have the submitted services compete in some "bot wars."
Last Week's Fragment - Solution
Last week's puzzle continued with our simple RPS game:
Write code to score the results of calling rock, paper, scissors (RPS) web services. Remember, rock beats scissors, scissors beat paper, and paper beats rock. And bombs and dynamite don't count.
Here's another simple solution in PHP - my bot facing off against Spencer's:
<?php
$winners = array("rock" => "paper", "paper" => "scissors", "scissors" => "rock");
$url1 = 'http://writestreams.com/bots/rps';
$url2 = 'http://ninjatricks.net/rps.php';
$result1 = file_get_contents($url1);
$result2 = file_get_contents($url2);
printf("1: %s 2: %s\n", $result1, $result2);
if ($result1 == $result2)
echo "Tie";
elseif ($result1 == $winners[$result2])
echo "#1 wins!";
elseif ($result2 == $winners[$result1])
echo "#2 wins!";
else
echo "No winner. Someone's misbehaving.";
?>
Again, it's a bit oversimplified, but does the job.
Now you're ready for your first "bot war": see how your RPS bot competes with mine at http://writestreams.com.us/bots/rps.