The Friday Fragment
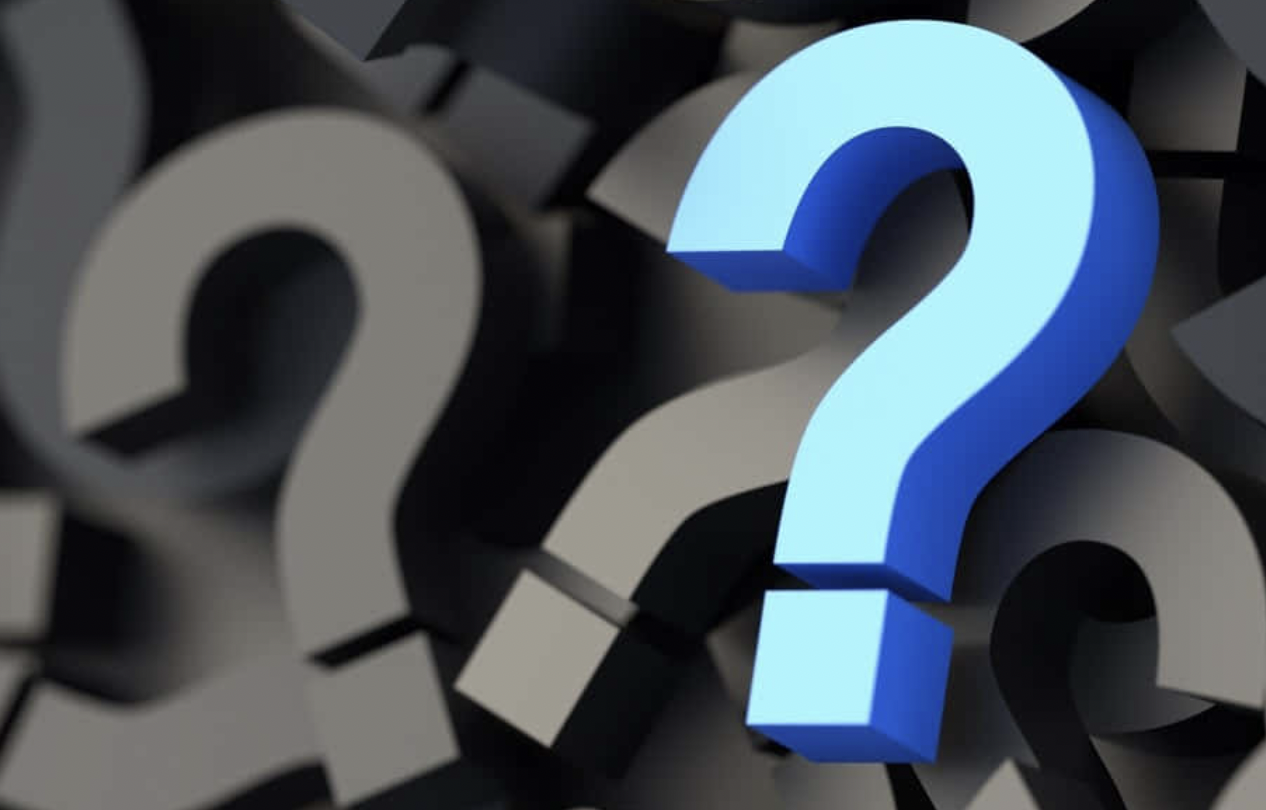
It's Friday, and time again for the Friday Fragment: our weekly programming-related puzzle.
This Week's Fragment
Even T-Rex likes to code games that play themselves, so let's continue with our Reversi "game bot:"
Write code to call competing Reversi bots, flip pieces, and score the results. If you'd like, you can build atop the solution we used for tic-tac-toe.
To “play along,” provide either the URL or the code for the solution. You can post it as a comment or send it via email.
Last Week's Fragment - Solution
Last week's puzzle was to start a Reversi (a.k.a., Othello) "bot:"
Code a simple REST web service to start playing Reversi. It should accept a 64-character string representing the current 8×8 “game board” with dot (empty space), X (black), or O (white) in each position (starting from the top left, going left-to-right and down). It should return a 64-character string with your next move added. Don’t worry about turning pieces yet, just find the next best move.
Here’s a simple solution in PHP, with room for improvement. To start playing, pass it ?board=...........................OX......XO...........................&piece=X.
<?php
if (!ereg('^[XO.]{64}$', $board=$_REQUEST['board']))
die(str_repeat('X',64));
if (!ereg('^[XO]$', $mypiece=$_REQUEST['piece']))
die(str_repeat('.',64));
if (!is_null($result = find_move($board, $mypiece)))
exit($result);
else
exit($board);
function find_move($board, $piece) {
STATIC $plays = array( 0, 7, 56, 63, // outer corners
2, 5, 16, 23, 40, 47, 58, 61, // A-squares
3, 4, 24, 32, 31, 39, 59, 60, // B-squares
18, 21, 42, 45, // inner corners
19, 20, 26, 29, 34, 37, 43, 44, // inner sides
10, 11, 12, 13, 17, 22, 25, 30, // mid sides
33, 38, 41, 46, 50, 51, 52, 53,
1, 6, 8, 15, 48, 55, 57, 62, // C-squares
9, 14, 49, 54 ); // X-squares
foreach ($plays as $play)
if ($board[$play] == '.' && can_play($board, $piece, $play)) {
$board[$play] = $piece;
return $board;
}
return null;
}
?>
Of course, there are much smarter ways to code Reversi, such as applying common strategies and running look-ahead scenarios, but that'd be more than a fragment. Years ago, a coworker wrote one for VisualWorks (still in the Cincom Public Repository), and there's a well-documented implementation at lurgee.net. If you're up for coding a smarter version behind the service interface, give it a shot.