The Friday Fragment
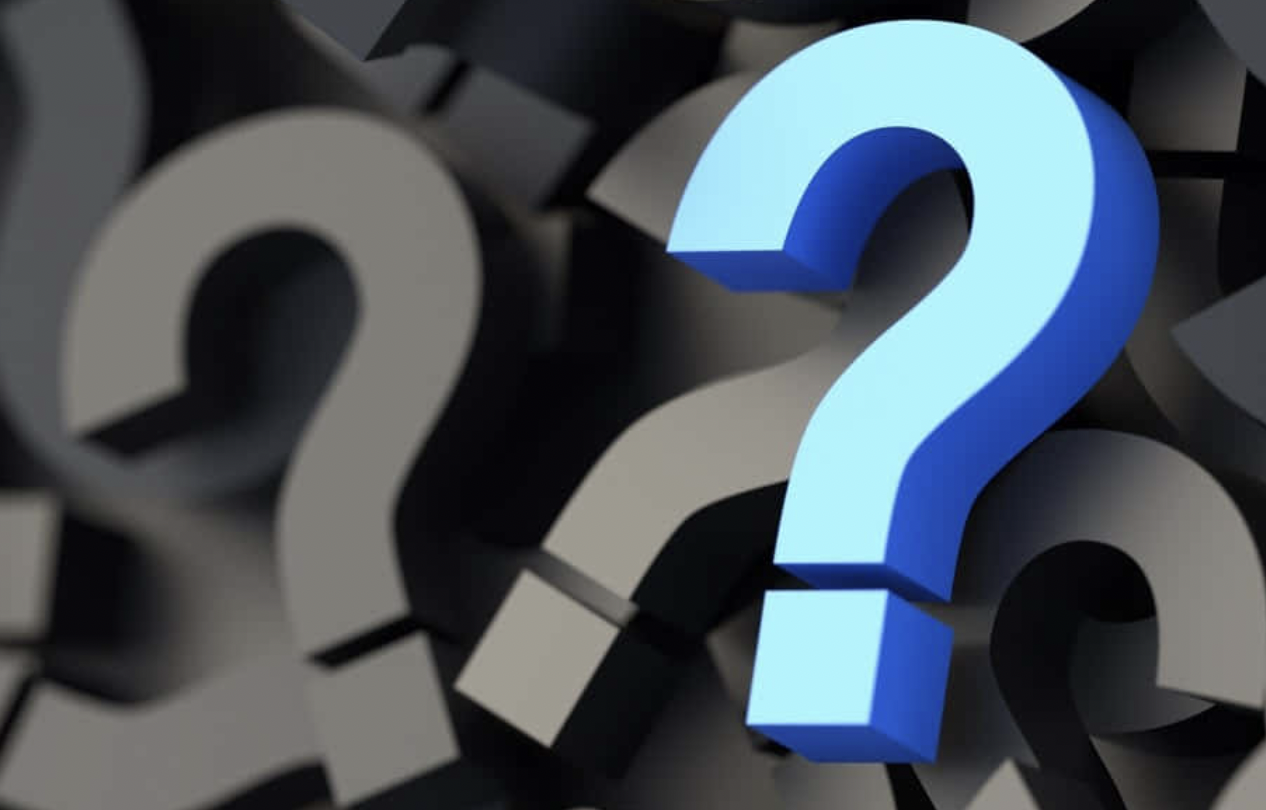
It's Friday, and time again for the Friday Fragment: our weekly programming-related puzzle.
This Week's Fragment
This year has Friday Fragments marking Christmas Eve and New Year's Eve. With that and another year change in mind, let's do a simple calendar-related puzzle:
Write code to calculate the day of week (Sunday through Saturday) for a given date. Remember to account for leap years.
To play along, post the code for the solution as a comment or send it via email.
Last Week's Fragment - Solution
With last week's puzzle, we conclude our round of game programming:
Upgrade your game (rock-paper-scissors, tic-tac-toe, or Reversi, your choice) to use async (AJAX-style) calls and updates, rather than postbacks. Use any frameworks you'd like, or just plain old Javascript.
My Reversi solution is at http://writestreams.com/bots/reversi/reversiajax.php. I used jQuery for the AJAX (get) calls, and ported much of playreversi.php and reversimove.php to Javascript. Here's the gist of it:
var url = "http://writestreams.com/bots/reversi"; // Your bot here
var board = "...........................OX..." +
"...XO...........................";
function userMove(row, col) {
var index = (row*8) + col;
var play = board.substr(0, index) + "X" + board.substr(index + 1);
board = updateBoard(board, play);
if (board.indexOf(".") >= 0)
callBot();
else
showBoard();
}
function callBot() {
var fullUrl = url + "?board=" + board + "&piece=O";
$.get(fullUrl, "", function(play) { botReturn(play) }, "text");
}
function botReturn(play) {
board = updateBoard(board, play);
showBoard();
}
function showBoard() {
var boardTable = document.getElementById("boardTable");
for (var row=0; row<8; row++)
for (var col=0; col<8; col++) {
var piece = board.charAt((row*8)+col);
var cellContents = "<img src='" + imageForPiece(piece) + "'>";
if (piece == "." && canPlay(board, "X", (row*8)+col))
cellContents = "<a href='javascript:userMove(" + row + "," + col + ")'>"
+ cellContents + "</a>";
boardTable.rows[row].cells[col].innerHTML = cellContents;
}
}
To see the helper functions, view the Javascript source. I continue to call my reversi web service bot; you can substitute your own at the "Your bot here" line.
By moving most of the behavior to the client, I greatly simplified the server-side PHP code (see the reversiajax.php source code). You can view all our games and bots at http://writestreams.com/bots.